API Dependencies Driving You Crazy? Try This Simple Mocking Solution
Using Wiremock, Swagger, Studio and Docker
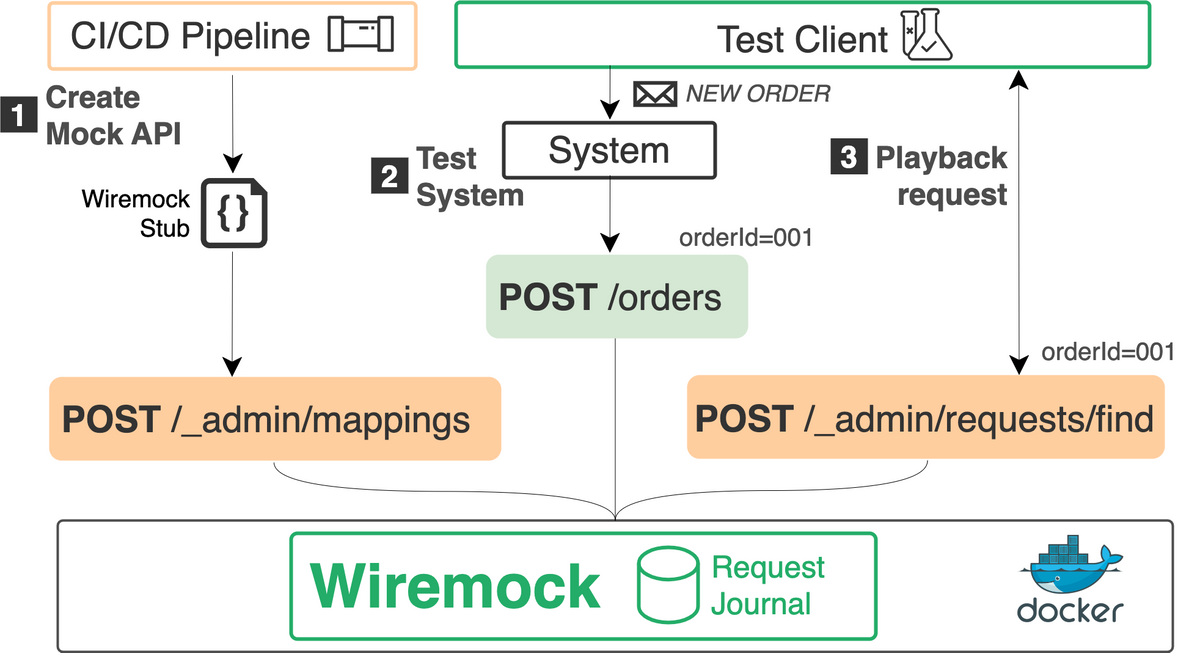
Are you looking for an API mocking solution where you can:
- Define mocking rules using configuration (no code)
- Convert Swagger definitions into mock APIs
- Record and playback requests captured by the mock API - programmatically
- Managed and deploy mock API configurations using CI/CD
- Deploy locally or to the cloud using Docker
If the answer is yes, keep reading.
Why do we need mock APIs?
If you're working with APIs, at some point, you'll become blocked. It's inevitable.
Perhaps the API isn't built. The test environment is unstable. Or, the API is an external dependency where you cannot test all scenarios.
The solution: mock the API.
Mock APIs allow you to build software components independently. They provide a means to test all scenarios by simulating API responses. Moreover, teams can write automated tests that call the mock server to verify:
- the client called the correct URI endpoint
- the client sent the correct number of requests; in the correct order
- and the request payload conforms to the agreed schema
What is Wiremock?
WireMock is a tool for simulating HTTP-based APIs. It allows developers to quickly mock and stub API endpoints, enabling them to simulate different scenarios and responses during testing and development processes.
How to run wiremock locally
Using the standalone JAR
Prerequisites: latest version of Java installed and installation location added to the PATH environment variable.
- Download the JAR from the wiremock site
- Run the command below in terminal to start the Wiremock service
java -jar wiremock-jre8-standalone-2.35.0.jar
Using Docker
Prerequisites: docker installed (obviously)
- Run the command below in terminal to create a new Docker container running Wiremock
docker run -it --rm -p 8080:8080 wiremock/wiremock
Converting OpenAPI specs into Wiremock stubs using Studio (optional)
The open-source version of Wiremock doesn’t provide a means to import OpenAPI specs.
Fortunately, a tool called Wiremock studio — albeit discontinued — converts OpenAPI specs to Wiremock stubs.
What is Studio?
Studio is a combination of Wiremock and a web-based authoring and management tool.
Please note: I don’t recommend hosting mock APIs within Studio as the product is discontinued. I use it purely as an authoring tool — converting OpenAPI specs to Wiremock stubs.
My workflow using Studio
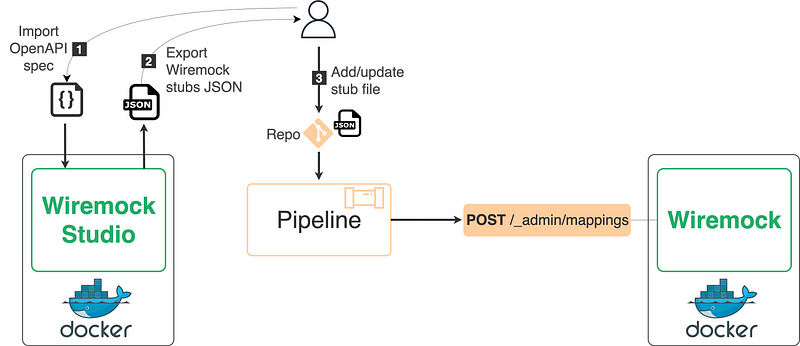
Running Wiremock Studio (requires Docker)
docker run -it --rm -p 9000:9000 up9inc/wiremock-studio
If it works you should see something like this in terminal:
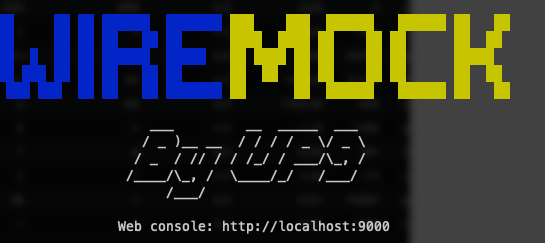
Importing an OpenAPI spec into Studio
- Open the browser and go to http://localhost:9000.
- Click ‘create new mock’.
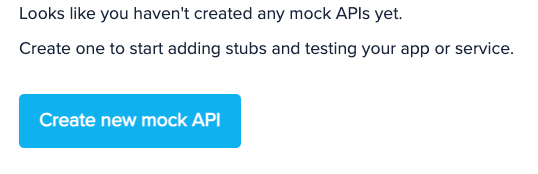
3. On the ‘Create new mock API’ screen select ‘blank’.
4. Enter a name for the API and click save.
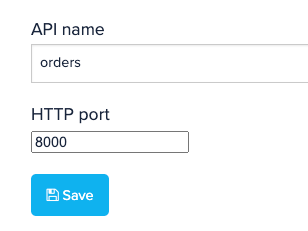
5. Click Stubs at the top left menu
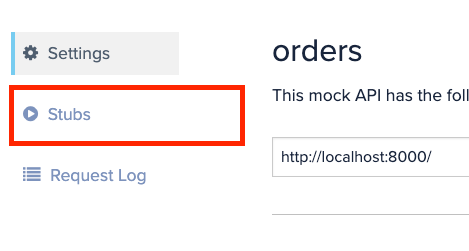
6. Click import

7. Select text.
8. Copy and paste the contents of the Swagger spec into the text box and click import
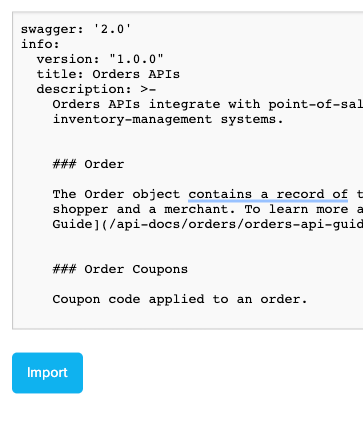
Exporting the stubs into a Wiremock JSON file
- While in the stubs area of the API, click Export (top right of the ribbon)

Using Wiremock’s request journal: verifying code is doing what it’s supposed to
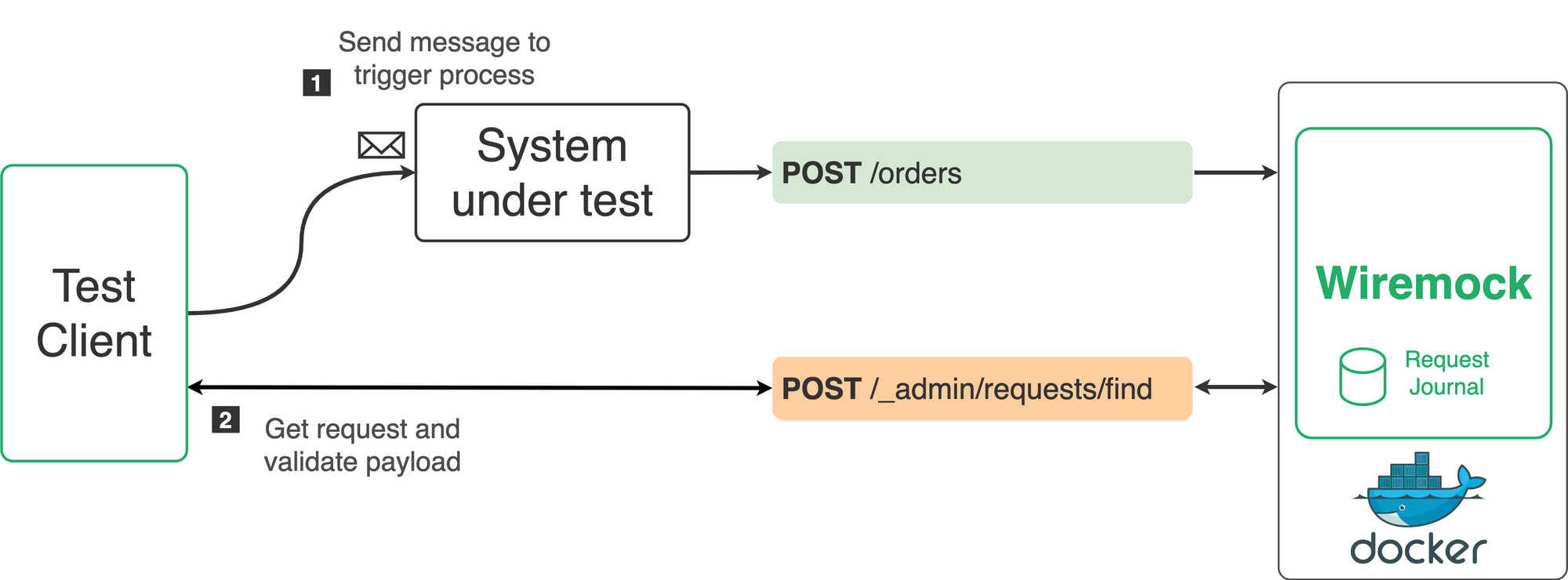
Wiremock records all received HTTP requests (known as recording).
The HTTP requests are stored in the request journal — an in-memory store of captured requests. Think of it as a transaction log.
You can access the request journal programmatically using Wiremock's management REST API.
Click here to view the official API documentation.
Using Wiremock's playback feature, we verified all known integration scenarios without calling the actual API.
As you see in the diagram above, our test cases would send a message into the system under test, which would call the mock API. Once the test case had submitted the message, we used Wiremock's REST API to retrieve the raw request and run a series of assertions.
To view all requests in the journal
curl --location --request GET '<URL>:8000/__admin/requests' \
--header 'Content-Type: application/json'
To find a specific request e.g., orderId:001
curl --location --request POST '<URL>:8000/__admin/requests/find' \
--header 'Content-Type: application/json' \
--data-raw '{
"method": "POST",
"url": "/orders",
"bodyPatterns": [
{"matchesJsonPath": "$..[?(@.orderId == '\''001'\'')]"}
]
}
Final thoughts
Wiremock and Docker are a great combo. They’re cheap to run. Portable. And you can store the stub files in version control.
Storing the stubs in version control and using pull requests allowed us to confirm the mocks aligned with the integration logic.
Plus, we could verify our integration logic did what it was supposed to without waiting for the downstream API to be available.
I hope you found this interesting.